wraptimer¶
- 📖 Documentation
An amazing alternative to Python's builtin timeit
module that allows for high resolution timing of functions as well as in-depth line-by-line timing. It also exposes convenient classes to measure execution time for any arbitrary code.
Sync function example¶

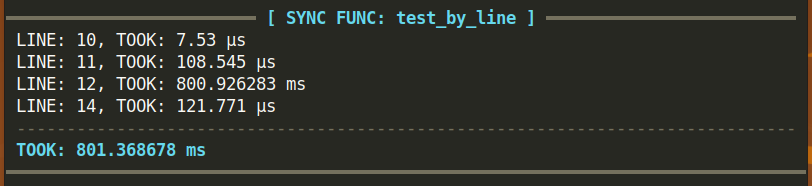
Async function example¶

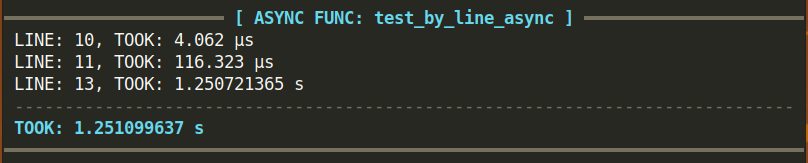
Don't need Line-by-line tracing?¶
Use @timeit.func
if you do not want to print line-by-line durations.
Read the documentation¶
For more about wraptimer, Read The Documentation